Model部分
实现INotifyPropertyChanged接口,使其能够发出某一属性值发生改变的通知
using System;
using System.Collections.Generic;
using System.ComponentModel;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
namespace MVVMTest.Model
public class PersonModel : INotifyPropertyChanged
public event PropertyChangedEventHandler PropertyChanged;
public PersonModel()
Name = "name";
Age = "20";
Sex = "男";
public string name;
public string Name
get { return name;}
name = value;
PropertyChanged?.Invoke(this, new PropertyChangedEventArgs(Name));
public string age;
public string Age
get { return age; }
age = value;
PropertyChanged?.Invoke(this, new PropertyChangedEventArgs("Age"));
public string sex;
public string Sex
get { return sex; }
sex = value;
PropertyChanged?.Invoke(this, new PropertyChangedEventArgs("Sex"));
ViewModel部分:
首先实现Icommand接口,包含一个Action和Func委托。
public class DeleteCommand<T> : ICommand
public event EventHandler CanExecuteChanged;
public Action<T> _execute = null;
public Func<T, bool> _canExecute = null ;
public DeleteCommand(Action<T> execute ):this(execute,null)
public DeleteCommand(Action<T> execute, Func<T, bool> canExecute)
if (canExecute != null)
this._canExecute = new Func<T, bool>(canExecute);
if (execute != null)
this._execute = new Action<T>(execute);
public void RaiseCanExceuteChanged()
if (CanExecuteChanged != null)
CanExecuteChanged(this, EventArgs.Empty);
public bool CanExecute(object parameter)
if (this._canExecute == null)
return true;
return _canExecute((T)(object)(parameter));
public void Execute(object parameter)
this._execute((T)(object)(parameter));
PersonViewModel类作为数据源,其中包含Model实例和一个DeleteCommand命令;
public class PersonViewModel
// public delegate void Fun(PersonModel person);
public PersonModel Model{ set; get; }
public DeleteCommand<PersonModel> deleteCommand { set; get; }
public PersonViewModel()
Model = new PersonModel();
deleteCommand = new DeleteCommand<PersonModel>( ClearData ,IsPersonNotEmpty);
public void ClearData(PersonModel person)
// PersonModel personModel = person as PersonModel;
Model.Age = "";
Model.Name = "";
Model.Sex = "";
public bool IsPersonNotEmpty(PersonModel person)
// PersonModel personModel = person as PersonModel;
// if (string.IsNullOrEmpty(personModel.Name) && string.IsNullOrEmpty(personModel.Age) && string.IsNullOrEmpty(personModel.Sex))
// return false;
return true;
View部分
<Window x:Class="MVVMTest.MainWindow"
xmlns="http://schemas.microsoft.com/winfx/2006/xaml/presentation"
xmlns:x="http://schemas.microsoft.com/winfx/2006/xaml"
xmlns:d="http://schemas.microsoft.com/expression/blend/2008"
xmlns:mc="http://schemas.openxmlformats.org/markup-compatibility/2006"
xmlns:local="clr-namespace:MVVMTest"
mc:Ignorable="d"
Title="MainWindow" Height="200" Width="525">
<Grid Margin="0,0,0,0">
<StackPanel Margin="0,0,0,0">
<StackPanel Orientation="Horizontal">
<TextBlock Text="Name" Width="100" Margin="10" TextAlignment="Center" VerticalAlignment="Center" HorizontalAlignment="Center"/>
<TextBox Width="100" Text="{Binding Model.Name}" Height="30" Margin="10" />
<Button x:Name="btn" Content="Clear" Command="{Binding deleteCommand}" Width="100" Height="30" Margin="150,10,10,10"/>
</StackPanel>
<StackPanel Orientation="Horizontal">
<TextBlock Text="Age" Width="100" Margin="10" TextAlignment="Center" VerticalAlignment="Center" HorizontalAlignment="Center"/>
<TextBox Width="100" Text="{Binding Model.Age}" Height="30" Margin="10"/>
</StackPanel>
<StackPanel Orientation="Horizontal">
<TextBlock Text="Sex" Width="100" Margin="10" TextAlignment="Center" VerticalAlignment="Center" HorizontalAlignment="Center"/>
<TextBox Width="100" Text="{Binding Model.Sex}" Height="30" Margin="10"/>
</StackPanel>
</StackPanel>
</Grid>
</Window>
程序运行:
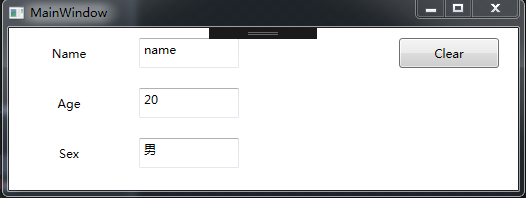
点击Clear按钮
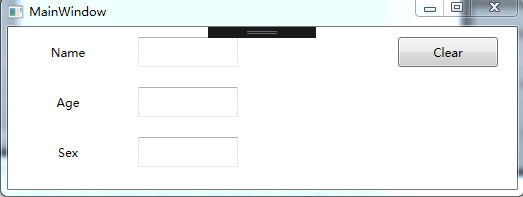
MVVM框架的目的就是让视图和业务逻辑分离,各干各的。那么怎样实现分离呢,精髓就是绑定ICommand。先看一下ICommand接口的定义:
// 摘要:
// 定义一个命令
[TypeConverter("System.Windows.Input.CommandConverter, PresentationFramework, Version=4.0.0.0, Cult
有这样一个可编程的新型遥控器, 它有7个可编程插槽, 每个插槽可连接不同的家用电器设备. 每个插槽对应两个按钮: 开, 关(ON, OFF). 此外还有一个全局的取消按钮(UNDO).
现在客户想使用这个遥控器来控制不同厂家的家用电器, 例如电灯, 热水器, 风扇, 音响等等.
客户提出让我编写一个接口, 可以让这个遥控器控制插在插槽...
Reproduced:https://www.codeproject.com/Articles/1052346/ICommand-Interface-in-WPF Author: Snesh Prajapati---------------------------------------IntroductionIn this article, we will learn about IComman...
命令第一个用途是将语义以及调用命令的对象与执行命令的逻辑分离开来。
WPF 命令中的四个主要概念:
WPF 中的路由命令模型可以分为四个主要概念:命令、命令源、命令目标以及命令绑定:
命令是要执行的操作。
命令源是调用命令的对象。
命令目标是在其上执行命令的对象。
命令绑定是将命令逻辑映射到命令的对象。
WPF 中的命令是通过实现 ICommand 接...
在学习MVVM的过程中,用到了ICommand这个重要的消息模式,不过都是看的前辈们的代码去模仿。
我想,学习不仅要学技能,更要学原理。
当我们谈及Commands时,一般说来,Command有两个功能:
a:执行一个特殊的行为:command的主要功能。
b:确定某一UIElement的视觉状态(visual state):例如确定button是否可用。
我们如果需要在Command中传递参数,实现也很简单。DelegateCommand还有一个DelegateCommand版本,可以传递一个T类型的参数。
1.View的Button绑定,其中CommandParameter定义了一个“20”的参数
1、在MVVM中的Command命令:使用Command命令有四个步骤:创建命令、绑定命令、设置命令源、设置命令目标
2、在实现ViewModel时要引用两个公共类:ViewModelBase类 和 DelegateCommand类是必须要引用的,一般的情况下这两个类都是封装好的直接用就可以了。
① ViewModelBase:里面主要是封装一些公共的属性、构造函数和类的成员
② DelegateCommand:视图模型委托
命令是 Windows Presentation Foundation (WPF) 中的输入机制,它提供的输入处理比设备输入具有更高的语义级别。
命令有若干用途:
第一个用途是将语义以及调用命令的对象与执行命令的逻辑分离开来。这使得多个完全不同的源可以调用相同的命令逻辑,并使得可以针对不同的目标对命令逻辑进行自定义。
例如,在许多应用程序中都能找到的编辑操作 “复制”、 “剪切”和 “粘贴”都