public FutureTask(Callable<V> callable) {
if (callable == null)
throw new NullPointerException();
this.callable = callable;
this.state = NEW;
public FutureTask(Runnable runnable, V result) {
this.callable = Executors.callable(runnable, result);
this.state = NEW;
FutureTask 本身是一个任务,提供 run 方法的具体实现。run 方法会执行 callable.call()拿到任务结果,并将结果赋值给另一个属性 Object outcome。
当使用 get()方法获取异步任务结果时,其实就是等待任务 run 方法执行结束:在异步任务未结束前,生成等待节点,加入到等待队列,线程被挂起。如果异步任务执行结束后会给outcome赋值异步任务计算结果,并唤醒等待队列中的线程。
get()方法通过 FutureTask 的状态判断任务是否执行完成,如果是执行完成的状态,那么返回 outcome,如果线程被中断或者超时或者执行任务过程中抛出异常则 get 方法抛出对应的异常。
FutureTask 的状态:
任务执行成功的状态变化示意图:
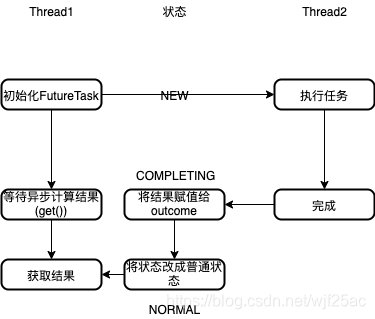
* state 表示 FutureTask 的状态。可能的状态一空有 7 种,初始化时是 NEW。状态变化的路径为:
* NEW -> COMPLETING -> NORMAL
* NEW -> COMPLETING -> EXCEPTIONAL
* NEW -> CANCELLED
* NEW -> INTERRUPTING -> INTERRUPTED
private volatile int state;
private static final int NEW = 0;
private static final int COMPLETING = 1;
private static final int NORMAL = 2;
private static final int EXCEPTIONAL = 3;
private static final int CANCELLED = 4;
private static final int INTERRUPTING = 5;
private static final int INTERRUPTED = 6;
private Callable<V> callable;
private Object outcome;
private volatile Thread runner;
private volatile WaitNode waiters;```
<a id="org00f89d1"></a>
### 构造方法
FutureTask 初始化需要外部指定的参数是 callable 对象,需要在执行任务时执行 callable.call 获取异步任务结果。所以构造方法都是围绕初始化 callable 对象而进行
```java
* 根据指定的 callable 对象生成一个 FutureTask 对象。
* @param callable callable 对象
* @throws NullPointerException 如果 callable 对象是空对象,那么抛出 NullPointerException 异常
public FutureTask(Callable<V> callable) {
if (callable == null)
throw new NullPointerException();
this.callable = callable;
this.state = NEW;
* 根据指定的 Runnable 对象生成 FutureTask。
* 借助 Executors public static <T> Callable<T> callable(Runnable task, T result)方法,利用 runnable 对象和泛型生成一个 callable 对象。
* @param runnable 可执行任务
* @param result callable 的执行结果的类型(执行 callable.call 的返回类型)
* @throws NullPointerException 如果 runnable 是空对象,那么抛出空指针异常
public FutureTask(Runnable runnable, V result) {
this.callable = Executors.callable(runnable, result);
this.state = NEW;
}```
<a id="org224cb8b"></a>
### Future 接口实现
```java
* 取消异步任务
* @param mayInterruptIfRunning 参数决定运行中的任务是否通过中断线程来取消任务。如果设置 false,那么运行中的任务无法取消。
* @return 如果取消成功则返回 true,否则返回 false。
public boolean cancel(boolean mayInterruptIfRunning) {
if (!(state == NEW &&
UNSAFE.compareAndSwapInt(this, stateOffset, NEW,
mayInterruptIfRunning ? INTERRUPTING : CANCELLED)))
return false;
try {
if (mayInterruptIfRunning) {
try {
Thread t = runner;
if (t != null)
t.interrupt();
} finally {
UNSAFE.putOrderedInt(this, stateOffset, INTERRUPTED);
} finally {
finishCompletion();
return true;
* FutureTask 的异步任务是否已经被取消
public boolean isCancelled() {
return state >= CANCELLED;
* 返回异步是否已经结束。非开始的状态都是结束的装填。
public boolean isDone() {
return state != NEW;
* 获取异步任务的计算结果
public V get() throws InterruptedException, ExecutionException {
int s = state;
if (s <= COMPLETING)
s = awaitDone(false, 0L);
return report(s);
* 可自定义超时时间的等待获取异步任务计算结果
public V get(long timeout, TimeUnit unit)
throws InterruptedException, ExecutionException, TimeoutException {
if (unit == null)
throw new NullPointerException();
int s = state;
if (s <= COMPLETING &&
(s = awaitDone(true, unit.toNanos(timeout))) <= COMPLETING)
throw new TimeoutException();
return report(s);
* 唤醒所有等待获取异步任务结果的线程
private void finishCompletion() {
for (WaitNode q; (q = waiters) != null;) {
if (UNSAFE.compareAndSwapObject(this, waitersOffset, q, null)) {
for (;;) {
Thread t = q.thread;
if (t != null) {
q.thread = null;
LockSupport.unpark(t);
WaitNode next = q.next;
if (next == null)
break;
q.next = null;
q = next;
break;
done();
callable = null;
* 等待异步任务结束
* @param timed 是否设置了超时时间
* @param nanos 超时时间
* @return state FutureTask 结束 awaitDone 时的的状态
private int awaitDone(boolean timed, long nanos)
throws InterruptedException {
final long deadline = timed ? System.nanoTime() + nanos : 0L;
WaitNode q = null;
boolean queued = false;
for (;;) {
if (Thread.interrupted()) {
removeWaiter(q);
throw new InterruptedException();
int s = state;
if (s > COMPLETING) {
if (q != null)
q.thread = null;
return s;
else if (s == COMPLETING)
Thread.yield();
else if (q == null)
q = new WaitNode();
else if (!queued)
queued = UNSAFE.compareAndSwapObject(this, waitersOffset,
q.next = waiters, q);
else if (timed) {
nanos = deadline - System.nanoTime();
if (nanos <= 0L) {
removeWaiter(q);
return state;
LockSupport.parkNanos(this, nanos);
LockSupport.park(this);
* 从阻塞队列中删除指定的等待节点
private void removeWaiter(WaitNode node) {
if (node != null) {
node.thread = null;
retry:
for (;;) {
for (WaitNode pred = null, q = waiters, s; q != null; q = s) {
s = q.next;
if (q.thread != null)
pred = q;
else if (pred != null) {
pred.next = s;
if (pred.thread == null)
continue retry;
else if (!UNSAFE.compareAndSwapObject(this, waitersOffset,
q, s))
continue retry;
break;