管理投影一般有两个操作:定义投影和转换投影
通常情况下导入数据一般自带投影,如果必须可以使用GeoSeries.set_crs()方法,然而我在运行时会报错’‘GeoDataFrame’ object has no attribute ‘set_crs’’,可以试试geodataframe.crs = {‘init’: ‘epsg:3395’},其中{‘init’: ‘epsg:3395’}表示墨卡托投影,任何能够被pyproj.CRS.from_user_input()语句接受的字符串,都能被geopandas正确使用其他比较常用的还有’epsg: 4326’表示wgs84投影,具体的坐标系代码可以通过www.spatialreference.org查阅。
对坐标系转换的方法可以使用geodataframe.to_crs(),我的版本会报错’‘b’no arguments in initialization list’,运行pyproj.Proj("+init=epsg:4326")发现不是pyproj库的问题,于是按照之前坐标系转换的方法做了一点修改{‘init’: ‘epsg:3395’},应当传入proj4字典,最新版本的可以直接使用,将wgs84转换为墨卡托坐标
world = world[(world.name != "Antarctica") & (world.name != "Fr. S. Antarctic Lands")]
world = world.to_crs({'init': 'epsg:3395'})
ax = world.plot()
ax.set_title("Mercator")
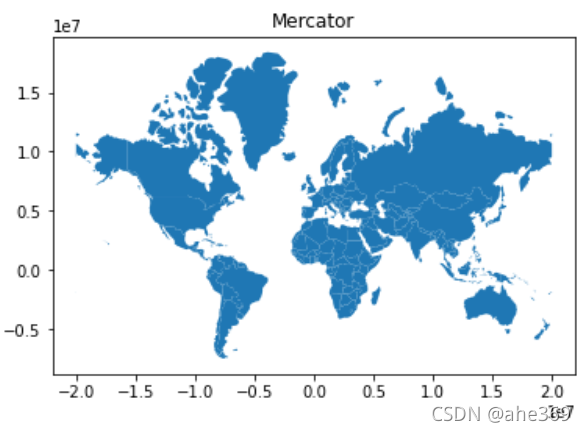
另外使用read_file()导入数据时,可以指定使用的坐标系,分为新的版本和旧的版本
GeoDataFrame(..., crs={'init': 'epsg:4326'})
gdf.crs = {'init': 'epsg:4326'}
gdf.to_crs({'init': 'epsg:4326'})
GeoDataFrame(..., crs="EPSG:4326")
gdf.crs = "EPSG:4326"
gdf.to_crs("EPSG:4326")
对Geopandas中的几何文件进行操作主要有如下几个方法
- GeoSeries.buffer(distance, resolution=16) #根据距离对图形扩展
- GeoSeries.boundary #以圆形向周围扩展
- GeoSeries.centroid #质心
- GeoSeries.convex_hull #变成凸多边形
- GeoSeries.envelope #返回点或者与坐标轴平行矩形
- GeoSeries.simplify(tolerance, preserve_topology=True) #对图形进行简化
- GeoSeries.unary_union #进行合并
下面对一些方法进行举例
import geopandas as gpd
from geopandas import GeoSeries
from shapely.geometry import Polygon
p1 = Polygon([(0, 0), (1, 0), (1, 1)])
p2 = Polygon([(0, 0), (1, 0), (1, 1), (0, 1)])
p3 = Polygon([(2, 0), (3, 0), (3, 1), (2, 1)])
g = GeoSeries([p2,p1, p3])
g.plot(cmap = 'GnBu')
buffer操作,注意画完图后坐标轴的范围
g.buffer(0.5)
g.buffer(0.5).plot(cmap = 'GnBu')
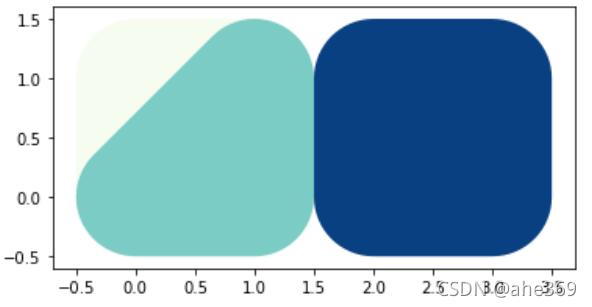
envelope操作,从结果可以看到,数据变成三个正方形了
g.envelope.plot(cmap = 'GnBu')
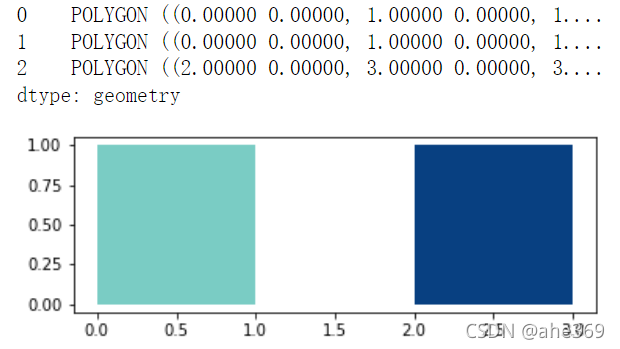
使用gdf.area()函数可以获取每个几何形状的面积
print(g.area)
nybb_path = gpd.datasets.get_path('nybb')
boros = gpd.read_file(nybb_path)
boros.set_index('BoroCode', inplace=True)
boros.sort_index(inplace=True)
boros.plot(cmap = 'GnBu')
boros['geometry'].convex_hull.plot()
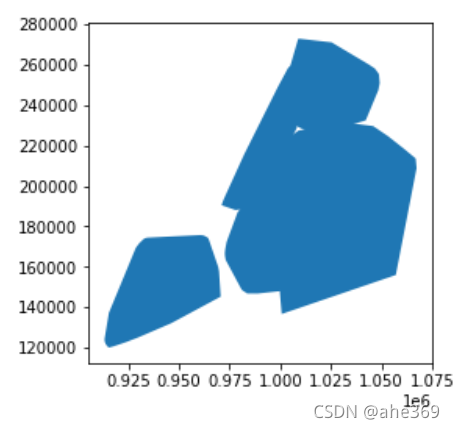
其他操作为,类似美图秀秀,对图片缩放、偏斜等等
GeoSeries.affine_transform(self, matrix)
GeoSeries.rotate(self, angle, origin='center', use_radians=False)
GeoSeries.scale(self, xfact=1.0, yfact=1.0, zfact=1.0, origin='center')
GeoSeries.skew(self, angle, origin='center', use_radians=False)
GeoSeries.translate(self, xoff=0.0, yoff=0.0, zoff=0.0)
根据重叠区域建立新的图形,主要使用函数为geodataframe.overlay()操作,具体的示意如下图
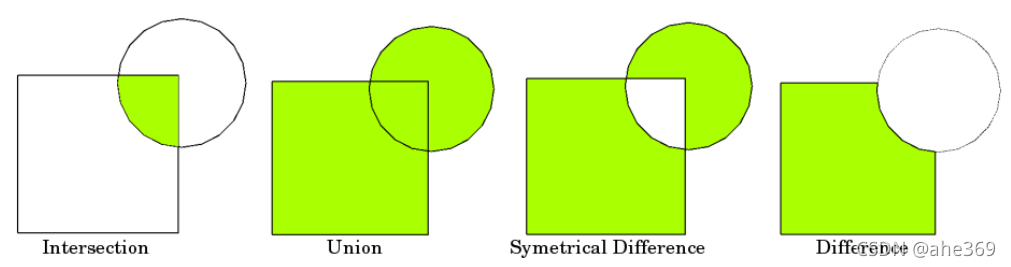
from shapely.geometry import Polygon
polys1 = geopandas.GeoSeries([Polygon([(0,0), (2,0), (2,2), (0,2)]),
Polygon([(2,2), (4,2), (4,4), (2,4)])])
polys2 = geopandas.GeoSeries([Polygon([(1,1), (3,1), (3,3), (1,3)]),
Polygon([(3,3), (5,3), (5,5), (3,5)])])
df1 = geopandas.GeoDataFrame({'geometry': polys1, 'df1':[1,2]})
df2 = geopandas.GeoDataFrame({'geometry': polys2, 'df2':[1,2]})
将例子画出来,原始的为两个形状红和绿,取并集后有多个图形,这里可以回顾一下多图层的画法
ax = df1.plot(color='red');
df2.plot(ax=ax, color='green', alpha=0.5)
res_union = gpd.overlay(df1,df2, how='union')
res_union.plot(cmap = 'GnBu')
res_intersection = gpd.overlay(df1, df2, how='intersection')
res_intersection.plot()
res_identity = gpd.overlay(df1, df2, how='identity')
res_identity
ax = res_identity.plot(cmap='tab10')
df1.plot(ax=ax, facecolor='none', edgecolor='k')
df2.plot(ax=ax, facecolor='none', edgecolor='k')
在非空间数据中,我们可以使用groupby()函数对数据进行汇总。在空间数据中,我们可以使用dissolve()函数聚合几何特征
world = geopandas.read_file(geopandas.datasets.get_path('naturalearth_lowres'))
world = world[['continent', 'geometry']]
continents = world.dissolve(by='continent')
continents.plot()
continents.head()
world = geopandas.read_file(geopandas.datasets.get_path('naturalearth_lowres'))
world = world[['continent', 'geometry', 'pop_est']]
continent = world.dissolve(by = 'continent', aggfunc = 'sum')
continent.plot(column = 'pop_est', scheme='quantiles', cmap='YlOrRd')
连接主要分为属性连接(attribute joins)和空间连接(spatial joins)
world = geopandas.read_file(geopandas.datasets.get_path('naturalearth_lowres'))
cities = geopandas.read_file(geopandas.datasets.get_path('naturalearth_cities'))
country_shapes = world[['geometry', 'iso_a3']]
country_names = world[['name', 'iso_a3']]
countries = world[['geometry', 'name']]
countries = countries.rename(columns={'name':'country'})
属性连接主要使用geodataframe.merge()方法,和pandas的merge()用法相似,如果使用pandas.merge()对GeoDateFrame方法进行连接,则返回结果不再是GeoDataFrame数据
country_shapes = country_shapes.merge(country_names, on = 'iso_a3')
country_shapes.head()
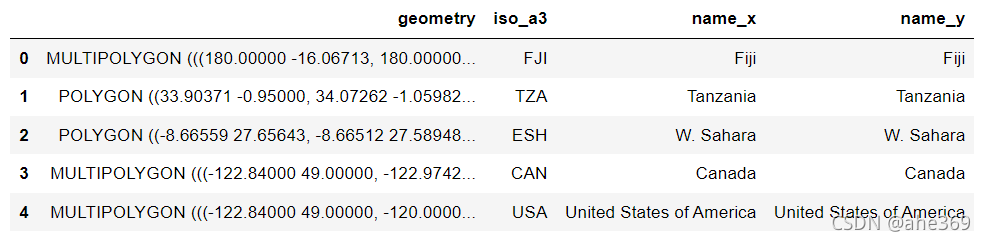
空间链接,使用gdf.sjoin()函数,spacial joins两个Geometry数据的连接,基于他们的空间关系
countries.head()
cities.head()
cities_with_country = gpd.sjoin(cities, countries, how="inner")
Geopandas提供了两个空间连接函数
- gpd.sjoin(),基于binary predicates (intersects, contains, etc.)二进制谓词
- 和gpd.sjoin_nearest(), 基于邻进度,可以设置最大搜索半径
- gpd.sjoin()有两个核心how 和 predicate
- predicate: intersects\contains\within\touches\crosses\overlaps
- how:left\right\inner,其中inner使用共同的索引值,并且只保留左边的Geodf
另外使用gdf.append()可以对gdf数据进行拼接,类似pandas中的append()方法。同理pd.concat([])方法也可以使用
joined = world.geometry.append(cities.geometry)
pd.concat([world, cities])
以上就是对GeoPandas库简单学习过程,该库可以和OSMnx结合,从OSMnx库获取地图资源,再通过GeoPandas进行可视化,以后有机会可以分享一下OSMnx的学习过程。下面为通过GeoPandas库制作的效果图
Geopandas官网(https://geopandas.org/en/stable/index.html)
GeoPandas库使用入门教程Geopandas入门前言Geopandas库扩展了Pandas的内容,使Python可以对几何类型进行空间操作,其大部分用法同Pandas一致。在教程开始之前,导入最基本的库import geopandas as gpdimport pandas as pdimport matplotlib.pyplot as plt使用步骤1.文件导入\导出与数据结构使用read_file()可以导入任何的空间矢量数据,主要有shp和GeoJson两种。具体用法为ge
本快速教程介绍了GeoPandas的主要概念和基本功能,以帮助您开始编写您的项目。
GeoPandas,顾名思义,通过增加对地理空间数据的支持,扩展了流行的数据科学库pandas 如果你对 pandas不熟悉,我们建议在继续之前快速浏览一下它的快速入门文档。
GeoPandas的核心数据结构是g
python-geopandas读取、创建shapefile文件、geopandas学习教程
shapefile是GIS中非常重要的一种数据类型,在ArcGIS中被称为要素类(Feature Class),主要包括点(point)、线(polyline)和多边形(polygon)。作为一种十分常见的矢量文件格式,geopandas对shapefile提供了很好的读取和写出支持 。
geopandas库允许对几何类型进行空间操作,其DataFrame结构相当于GIS数据中的一张属性表,使得可以直接操作矢量数据
GeoPandas是一个开源项目,可以更轻松地使用python处理地理空间数据。
GeoPandas扩展了Pandas中使用的数据类型DataFrame,允许对几何类型进行空间操作。
GeoPandas的目标是使在python中使用地理空间数据更容易。它结合了Pandas和Shapely的能力,提供了Pandas的地理空间操作和多种Shapely的高级接口。GeoPandas可以让您轻松地在python中进行操作,否则将需要...
安装Geopandas的附带库 fiona、gdal、pyproj、shapely,
所以可参考以下步骤操作,以及我的python版本为3.7:下载地址:https://www.lfd.uci.edu/~gohlke/pythonlibs/#gdal
下载到自己的python安装目录下的pip文件夹中。注意whl文件版本号(cp36、cp37等)要与自己电脑匹配,与我电脑匹配的是cp37。
运行cmd——转到whl文件所在目录下——输入pip install whl文件名。
将以上4个whl文件安装成功之后,用清华镜像网址直接安装:pip install -i https://pypi.tuna.tsinghua.edu.cn/simple geopandas
包含下载好的 Fiona , GDAl , pyproj , Shapely whl文件(py3.7,win64)
其他包可以通过 pip install geopandas 安装,步骤为cd到指定目录下:
1.pip install 安装GDAL-3.0.3-cp37-cp37m-win_amd64.whl
2.pip install 安装Shapely-1.6.4.post2-cp37-cp37m-win_amd64.whl
3.pip install 安装Fiona-1.8.13-cp37-cp37m-win_amd64.whl
4.pip install 安装pyproj-2.4.2.post1-cp37-cp37m-win_amd64.whl
5.pip install 安装geopandas-0.6.2-py2.py3-none-any.whl,即可成功安装geopandas!
由于geopandas库是基于pandas和shapely库的二次开发,因此在下载geopandas之前,需要先安装好这两个库。
1. 安装pandas库
可以在命令行中使用pip命令来安装pandas库:
pip install pandas
2. 安装shapely库
同样可以使用pip命令来安装shapely库:
pip install shapely
3. 安装geopandas库
安装好pandas和shapely库后,就可以使用pip命令来安装geopandas库了:
pip install geopandas
安装好之后,就可以在python代码中使用geopandas库了。