UE4-XML文件读写
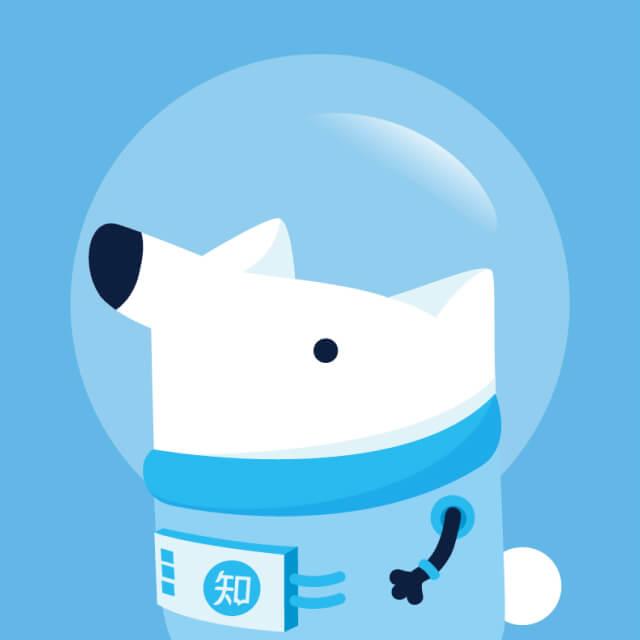

UE4自带一个XmlParser,可以很方便的实现Xml的简单读写功能。
- 在PublicDependencyModuleNames.AddRange中添加XmlParser。
- 在文件中添加#include “XmlParser\Public\XmlParser.h"
其主要功能包括节点值读取和节点属性读取,仅提供了设置节点值和添加节点的功能,没有提供属性设置功能,不能创建跟节点。
1)主要功能
class XMLPARSER_API FXmlFile
public:
FXmlFile() : RootNode(nullptr), bFileLoaded(false) {}
FXmlFile(const FString& InFile, EConstructMethod::Type ConstructMethod = EConstructMethod::ConstructFromFile);
~FXmlFile() { Clear(); };
private:
FXmlFile(const FXmlFile& rhs) {}
FXmlFile& operator=(const FXmlFile& rhs) { return *this; };
public:
//加载文件
bool LoadFile(const FString& Path, EConstructMethod::Type ConstructMethod = EConstructMethod::ConstructFromFile);
FString GetLastError() const;//获取最后一条错误消息
void Clear();//清除所有内部文件。注意:使指向FXmlNode的任何现有指针无效
/** Checks to see if a file is loaded */
bool IsValid() const;//判断文件是否加载成功
const FXmlNode* GetRootNode() const;//返回跟节点
FXmlNode* GetRootNode();//返回跟节点
bool Save(const FString& Path);//写入磁盘,目前仅支持UTF-16格式
private:
private:
FString LoadedFile;//文件路径
FString ErrorMessage;//返回给客户端的错误消息
FXmlNode* RootNode;//跟节点指针
bool bFileLoaded;//文件是否被加载
bool bCreationFailed;//节点创建过程是否失败
};
xmlnode的主要功能
class XMLPARSER_API FXmlAttribute
public:
FXmlAttribute(const FString& InTag, const FString& InValue)
: Tag(InTag)
, Value(InValue)
const FString& GetTag() const;//读取属性名称
const FString& GetValue() const;//读取属性值
private:
FString Tag;//属性名称
FString Value;//属性值
class XMLPARSER_API FXmlNode
friend class FXmlFile;
private:
FXmlNode() : NextNode(nullptr) {}
FXmlNode(const FXmlNode& rhs) {}
~FXmlNode() { Delete(); }
void Delete();
public:
const FXmlNode* GetNextNode() const;//获得下一个节点
const TArray<FXmlNode*>& GetChildrenNodes() const;//获得所有子节点
const FXmlNode* GetFirstChildNode() const;//获得第一个子节点
const FXmlNode* FindChildNode(const FString& InTag) const;//根据节点名称查找子节点
FXmlNode* FindChildNode(const FString& InTag);//
const FString& GetTag() const;//获得节点名称
const FString& GetContent() const;//获得节点值
const TArray<FXmlAttribute>& GetAttributes() const;//获得节点属性列表
FString GetAttribute(const FString& InTag) const;//获得节点某个属性
void SetContent(const FString& InContent);//接入节点值
void AppendChildNode(const FString& InTag, const FString& InContent);//添加子节点
private:
TArray<FXmlNode*> Children;//子节点列表
TArray<FXmlAttribute> Attributes;//节点属性列表
FString Tag;//节点名称
FString Content;//节点内容
FXmlNode* NextNode;//下一个节点指针
};
2)示例
- XML文件
<?xml version="1.0" ?>
<list id="0">
<item id="1" property="静态文本框1" text="HelloWorld_1" variable="string" type="STextBlock"/>
<item id="2" property="TestString_2" text="HelloWorld_2" variable="string" type="STextBlock"/>
</list>
-文件读写
//读取文件
FString filePath = TEXT("C:\\git\\list.fig.xml");
FXmlFile xml(filePath);
if (!xml.IsValid())
return;
//获取顶级节点
FXmlNode * topNode = xml.GetRootNode();
//获取所有子节点
const TArray<FXmlNode*>& children = topNode->GetChildrenNodes();
//获取第一个子节点的属性
FString value = topNode->GetFirstChildNode()->GetAttribute(TEXT("property"));
//写入值
topNode->AppendChildNode(TEXT("testNode"),TEXT("haha"));
xml.Save(TEXT("C:\\git\\testSave.xml"));
通过TinyXML实现复杂的XML读写功能
github下载tinyxml2相关文件,包括tinyxml2.h和tinyxml2.cpp
头文件中引入#include "tinyxml2.h" 及 using namespace tinyxml2;
UE4在Windows下FString默认为TChar,在使用tinyxml时,通过TCHAR_TO_UTF8实现FString到std::string的转换,通过UTF8_TO_TCHAR实现std::string到FString的转换,同时保存文件时,应确保文件采用UTF8文件格式。
- XML写入文件
XMLDocument * doc = new XMLDocument;
XMLDeclaration *declaration = doc->NewDeclaration("xml version=\"1.0\" encoding=\"UTF-8\"");
doc->LinkEndChild(declaration);
XMLElement *School = doc->NewElement(TCHAR_TO_UTF8(TEXT("学校")));
doc->LinkEndChild(School);
School->SetAttribute(TCHAR_TO_UTF8(TEXT("名称")), TCHAR_TO_UTF8(TEXT("机械工程学院")));
XMLElement *Student = doc->NewElement(TCHAR_TO_UTF8(TEXT("学生")));
School->LinkEndChild(Student);
Student->SetAttribute(TCHAR_TO_UTF8(TEXT("姓名")), TCHAR_TO_UTF8(TEXT("天霸")));
Student->SetAttribute(TCHAR_TO_UTF8(TEXT("学号")), TCHAR_TO_UTF8(TEXT("01")));
if (XML_SUCCESS != doc->SaveFile(TCHAR_TO_UTF8(TEXT("C:\\git\\testSave.xml")))) {
delete doc;
delete doc;
-TinyXML读取文件
FString filePath = TEXT("C:\\git\\testSave.xml");
XMLDocument doc;
if (XML_SUCCESS != doc.LoadFile(TCHAR_TO_UTF8(*filePath))) {
return;
XMLElement * school = doc.RootElement();
if (school) {
XMLElement *node = school->FirstChildElement(TCHAR_TO_UTF8(TEXT("学生")));