C# 获取字典中最后一个元素
时间: 2024-03-12 16:49:06
浏览: 262
在 C# 中,字典是无序的,因此没有“最后一个”元素的概念。但是,你可以通过以下方式获取字典中的最后一个键值对:
```csharp
using System.Collections.Generic;
// ...
Dictionary<string, int> dict = new Dictionary<string, int>();
// 添加一些元素到字典中...
KeyValuePair<string, int> lastPair = dict.ElementAt(dict.Count - 1);
string lastKey = lastPair.Key;
int lastValue = lastPair.Value;
上述代码中,`ElementAt()` 方法可以通过索引获取字典中指定位置的键值对。由于字典是无序的,因此你需要使用 `Count - 1` 获取最后一个键值对。获取到最后一个键值对后,你可以获取其键和值。
相关问题
c#在使用字典时,如何获取字典中的元素插入的顺序
在 C# 中,标准的 Dictionary 类并不保证元素的插入顺序,因为它的设计目的是为了高效的查找和插入操作。如果你需要保持元素的插入顺序,可以考虑使用以下两种替代方案:
1. 使用 SortedDictionary 或者 ConcurrentSortedDictionary:
- SortedDictionary 继承自 Dictionary,并按照键的自然排序顺序维护元素。
- ConcurrentSortedDictionary 是线程安全的版本,可以在并发环境中使用。
```csharp
SortedDictionary<TKey, TValue> orderedDict = new SortedDictionary<TKey, TValue>();
orderedDict.Add("key", "value");
```
C# 获取字典目标位置的前一位
相关推荐
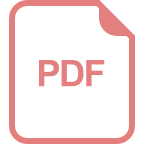
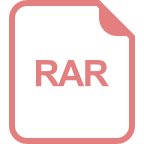
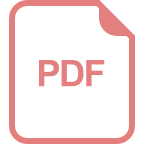
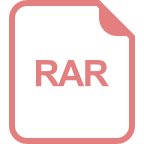
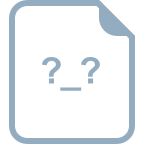
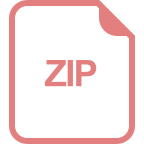
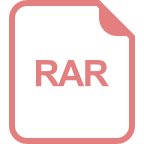
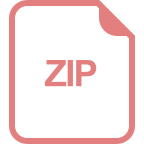






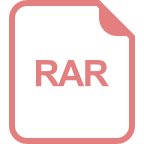
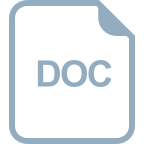