buffer 如何转化成MultipartFile
时间: 2023-10-24 13:32:38
浏览: 55
要将一个 `buffer` 转换为 `MultipartFile` [对象](https://geek.csdn.net/educolumn/04c51611e4b730957464192e0307b82c?spm=1055.2569.3001.10083),你可以使用 Python 的 `io.BytesIO` 类来创建一个类似文件的[对象](https://geek.csdn.net/educolumn/04c51611e4b730957464192e0307b82c?spm=1055.2569.3001.10083),并将 `buffer` 的内容写入该[对象](https://geek.csdn.net/educolumn/04c51611e4b730957464192e0307b82c?spm=1055.2569.3001.10083)。然后,你可以使用 `MultipartFile` 类的构造[函数](https://geek.csdn.net/educolumn/ba94496e6cfa8630df5d047358ad9719?dp_token=eyJ0eXAiOiJKV1QiLCJhbGciOiJIUzI1NiJ9.eyJpZCI6NDQ0MDg2MiwiZXhwIjoxNzA3MzcxOTM4LCJpYXQiOjE3MDY3NjcxMzgsInVzZXJuYW1lIjoid2VpeGluXzY4NjQ1NjQ1In0.RrTYEnMNYPC7AQdoij4SBb0kKEgHoyvF-bZOG2eGQvc&spm=1055.2569.3001.10083)来创建一个 `MultipartFile` 对象。下面是一个示例代码:
```python
import io
from django.core.files import File
def buffer_to_multipart_file(buffer):
# 创建一个 BytesIO 对象
file_object = io.BytesIO()
# 将 buffer 写入 BytesIO 对象
file_object.write(buffer)
# 将 BytesIO 对象转换为 MultipartFile 对象
multipart_file = File(file_object)
return multipart_file
在这个示例中,我们使用了 Django 的 `File` 类来创建一个 `MultipartFile` 对象。如果你正在使用其他框架或库,你需要根据其具体[文档](https://geek.csdn.net/edu/300981ef51993cfd737d329c71ba77f9?dp_token=eyJ0eXAiOiJKV1QiLCJhbGciOiJIUzI1NiJ9.eyJpZCI6NDQ0MDg2MiwiZXhwIjoxNzA3MzcxOTM4LCJpYXQiOjE3MDY3NjcxMzgsInVzZXJuYW1lIjoid2VpeGluXzY4NjQ1NjQ1In0.RrTYEnMNYPC7AQdoij4SBb0kKEgHoyvF-bZOG2eGQvc&spm=1055.2569.3001.10083)来创建对应的文件对象。记得将 `buffer` 替换为你实际的二进制数据。
相关问题
java 将url转化成MultipartFile
Java中将url转换为MultipartFile文件的过程需要经过以下几个步骤:
1. 通过URL对象获取文件的输入流。
2. 将输入流转换为字节数组。
3. 创建一个MockMultipartFile对象,并将字节数组、文件名、文件类型等信息传入其中。
下面是一个示例代码:
import java.io.ByteArrayOutputStream;
import java.io.InputStream;
import java.net.URL;
import org.springframework.mock.web.MockMultipartFile;
import org.springframework.web.multipart.MultipartFile;
public class UrlToMultipartFileUtil {
public static MultipartFile urlToMultipartFile(String urlStr) throws Exception {
URL url = new URL(urlStr);
InputStream inputStream = url.openStream();
ByteArrayOutputStream outputStream = new ByteArrayOutputStream();
byte[] buffer = new byte[1024];
int len;
while ((len = inputStream.read(buffer)) != -1) {
outputStream.write(buffer, 0, len);
byte[] bytes = outputStream.toByteArray();
inputStream.close();
outputStream.close();
MultipartFile multipartFile = new MockMultipartFile("file", bytes);
return multipartFile;
```
java把图片链接转换成MultipartFile
相关推荐
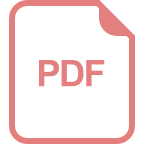
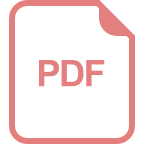












